Tutorial to create a serverless workflow using Lambda & Step Function
Tutorial Objectives:
1. Learn AWS Step Functions - a serverless orchestration service
2. Learn to create a Lambda Function
Step 1: Create a State Machine & Serverless Workflow
Log on to your AWS Management Console and open AWS Step Functions console and click on Get Started.
On the top left corner, click on State machines.
Now, click on Create State Machine.
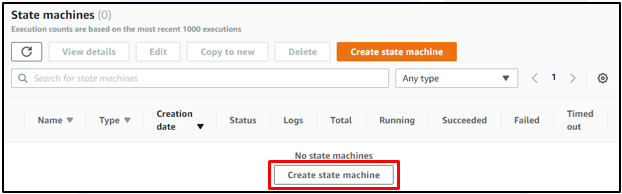
Under Define State Machine, choose Write your workflow in code.
Let the type remain as Standard.
Under Definition, replace the content with the following code.
Note: Replace ARN of your function name at the highlighted line below in your code.
{
"Comment": "A simple AWS Step Functions state machine that automates a call center support session.",
"StartAt": "Open Case",
"States": {
"Open Case": {
"Type": "Task",
"Resource": "arn:aws:lambda:REGION:ACCOUNT_ID:function:FUNCTION_NAME",
"Next": "Assign Case"
},
"Assign Case": {
"Type": "Task",
"Resource": "arn:aws:lambda:REGION:ACCOUNT_ID:function:FUNCTION_NAME",
"Next": "Work on Case"
},
"Work on Case": {
"Type": "Task",
"Resource": "arn:aws:lambda:REGION:ACCOUNT_ID:function:FUNCTION_NAME",
"Next": "Is Case Resolved"
},
"Is Case Resolved": {
"Type" : "Choice",
"Choices": [
{
"Variable": "$.Status",
"NumericEquals": 1,
"Next": "Close Case"
},
{
"Variable": "$.Status",
"NumericEquals": 0,
"Next": "Escalate Case"
}
]
},
"Close Case": {
"Type": "Task",
"Resource": "arn:aws:lambda:REGION:ACCOUNT_ID:function:FUNCTION_NAME",
"End": true
},
"Escalate Case": {
"Type": "Task",
"Resource": "arn:aws:lambda:REGION:ACCOUNT_ID:function:FUNCTION_NAME",
"Next": "Fail"
},
"Fail": {
"Type": "Fail",
"Cause": "Engage Tier 2 Support." }
}
}
Click the refresh button to show the state machine definition as a visual workflow.
Click Next.
Provide the name as CallCenterStateMachine.
Under Permissions, choose Create new role.
Keeping the rest as default, click on Create State Machine.
Step 2: Creating AWS Lambda Functions
Open AWS Management Console on a new tab and choose Lambda.
Click on Create Function.
Select Author from scratch.
Configure your first Lambda function with these settings:
· Name – OpenCaseFunction
· Runtime – Node.js 4.x
· Architecture – x86_64
Under Change Default execution role, choose Create a new role with basic Lambda permissions.
Click Create function.
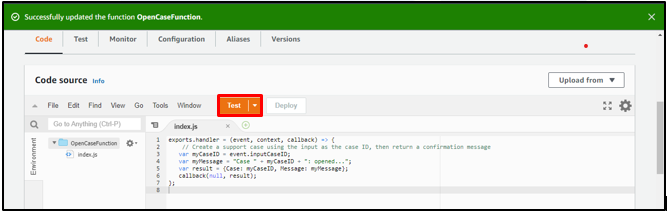
Under the Code segment, replace the present content with the following code and click on Deploy.
exports.handler = (event, context, callback) => {
// Create a support case using the input as the case ID, then return a confirmation message
var myCaseID = event.inputCaseID;
var myMessage = "Case " + myCaseID + ": opened...";
var result = {Case: myCaseID, Message: myMessage};
callback(null, result);
};
At the top of the page, click Functions.
Repeat previous steps from step 2 to create 4 more Lambda functions, using the OpenCaseFunction IAM role you created in the previous step.
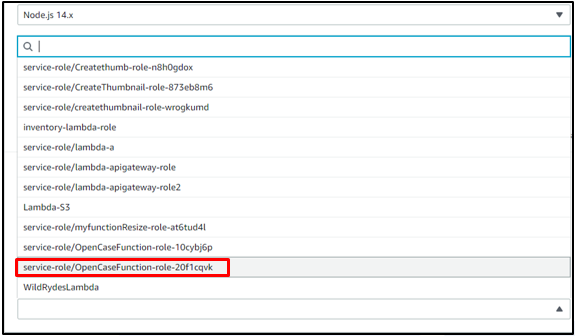
Define AssignCaseFunction as:
exports.handler = (event, context, callback) => {
// Assign the support case and update the status message
var myCaseID = event.Case;
var myMessage = event.Message + "assigned...";
var result = {Case: myCaseID, Message: myMessage};
callback(null, result);
};
Define WorkOnCaseFunction as:
exports.handler = (event, context, callback) => {
// Generate a random number to determine whether the support case has been resolved, then return that value along with the updated message.
var min = 0;
var max = 1;
var myCaseStatus = Math.floor(Math.random() * (max - min + 1)) + min;
var myCaseID = event.Case;
var myMessage = event.Message;
if (myCaseStatus == 1) {
// Support case has been resolved
myMessage = myMessage + "resolved...";
} else if (myCaseStatus == 0) {
// Support case is still open
myMessage = myMessage + "unresolved...";
}
var result = {Case: myCaseID, Status : myCaseStatus, Message: myMessage};
callback(null, result);
};
Define CloseCaseFunction as:
exports.handler = (event, context, callback) => {
// Close the support case
var myCaseStatus = event.Status;
var myCaseID = event.Case;
var myMessage = event.Message + "closed.";
var result = {Case: myCaseID, Status : myCaseStatus, Message: myMessage};
callback(null, result);
};
Define EscalateCaseFunction as:
exports.handler = (event, context, callback) => {
// Escalate the support case
var myCaseID = event.Case;
var myCaseStatus = event.Status;
var myMessage = event.Message + "escalating.";
var result = {Case: myCaseID, Status : myCaseStatus, Message: myMessage};
callback(null, result);
};
When complete, you should have 5 Lambda functions.
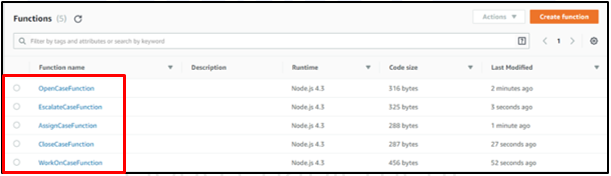
Step 3: Populate the workflow
Move back to the Step Functions Tab and on the State Machines page, select your CallCenterStateMachine and click Edit.
In the State machine definition section, find the line below the Open Case state which starts with Resource.
Replace the ARN with the ARN of your OpenCaseFunction.
If you click the sample ARN, a list of the AWS Lambda functions in your account will appear and you can select it from the list.
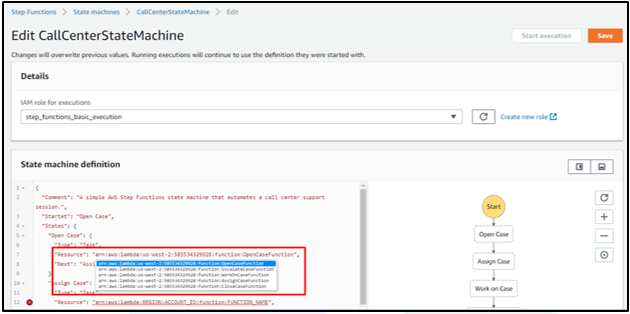
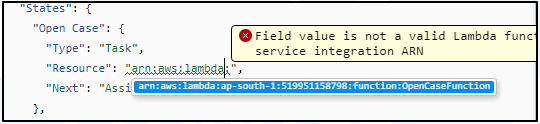
Repeat the previous step to update the Lambda function ARNs for the Assign Case, Work on Case, Close Case, and Escalate Case Task states in your state machine, then click Save.
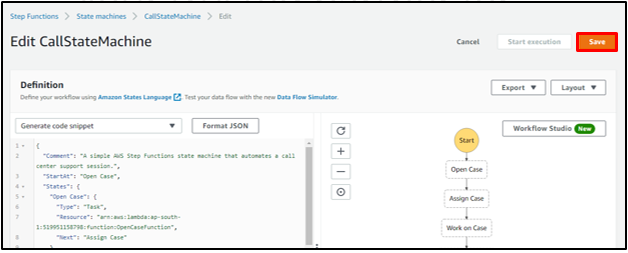
Step 4: Execute the workflow
Once saved, click on Start execution.
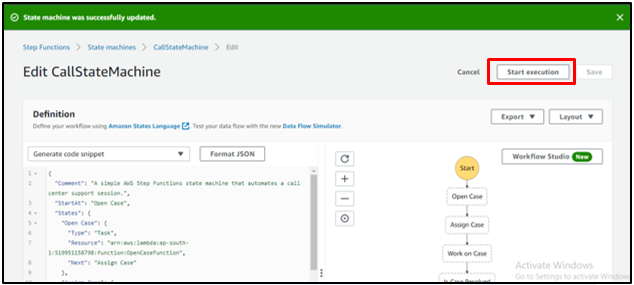
In the Input window, add the following code and click on Start execution.
{
"inputCaseID": "001"
}
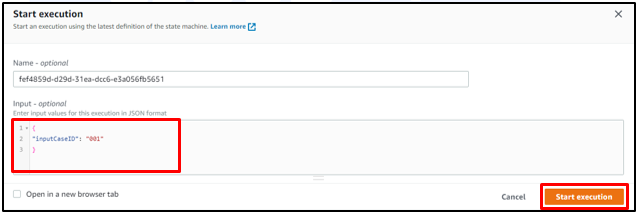
As your workflow executes, each step will change color in the Visual workflow pane. Wait a few seconds for execution to complete. Then, in the Execution details pane, click Input and Output to view the inputs and results of your workflow.
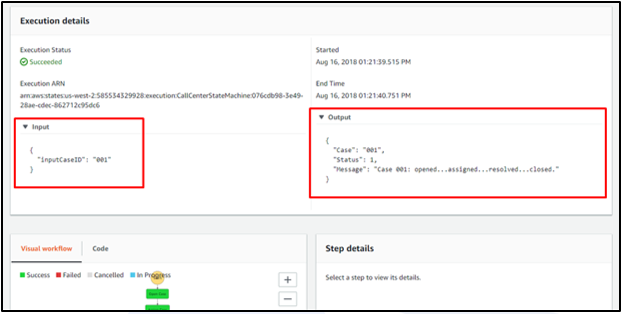
Scroll down to the Execution event history section. Click through each step of execution to see how Step Functions called your Lambda functions and passed data between functions.
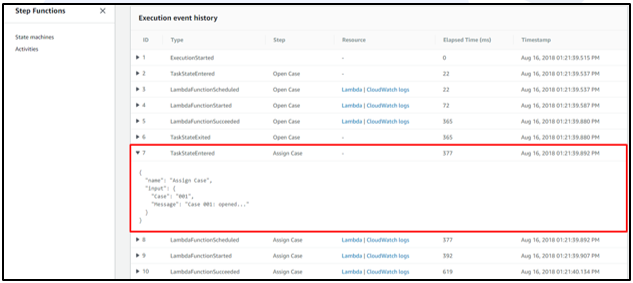
Depending on the output of your WorkOnCaseFunction, your workflow may have ended by resolving the support case and closing the ticket, or escalating the ticket to the next tier of support.
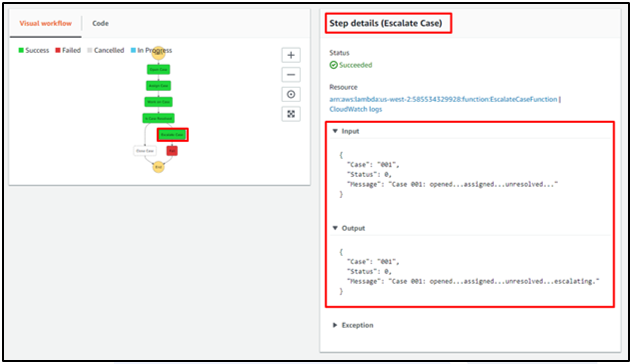
You can re-run the execution a few more times to observe this different behaviour. This image shows an execution of the workflow where the support case was escalated, causing the workflow to exit with a Fail state.
Note: Terminate the resources such as Lambda Functions, State Machines used if it is no longer needed.
Was this document helpful? How can we make this document better? Please provide your insights. You can download PDF version for reference.
We provide the best AWS training from Pune, India.
For AWS certification, contact us now.
This blog Easy to understand the concept of creating serverless workflow using Lambda
This blog is helps to understand the concept of creating serverless workflow using Lambda
creating a serverless workflow using Lambda & Step Function was understood
This tutorial is very clear and make things easy to understand.