Serverless workflow using AWS Step Functions to handle errors.
Tutorial Objectives:
1. Learn to use AWS Step Functions to handle errors in Serverless applications.
Step 1: Create an AWS Identity and Access Management (IAM) Role
Open the AWS Management Console, When the screen loads, enter your user name and password to get started. Next, Search for IAM, Go to roles and click on create role.
Type of Trusted entity: AWS Service
Choose a use case: Lambda
Next: Permissions, Next: tags, Next: Review
Role Name: lambda_basic_execution and click on Create role.
Similarly, create another role for step functions.
In another browser window, navigate to the AWS Management Console and type IAM in the search bar. Click IAM to open the service console.
Click Roles, then choose Create Role.
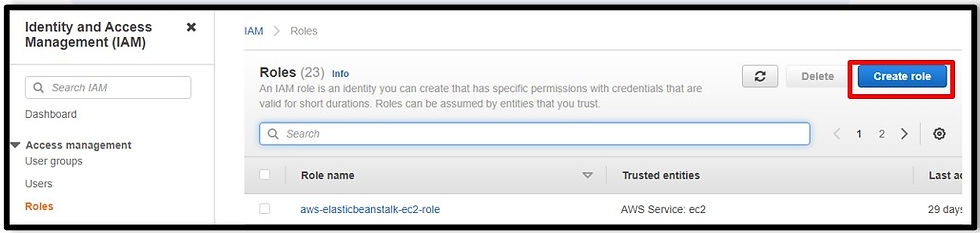
On the Select type of trusted entity page, under AWS service, select Step Functions from the list, and then choose Next: Permissions.

On the Attach permissions policy page, choose Next: Tags, Next: Review.
On the Review page, type step_functions_basic_execution for Role name and click Create role.

Your new IAM role is created and appears in the list beneath the IAM role for your Lambda function.
Step 2: Create Lambda Function.
In the new tab, open AWS console and type Lambda in the search bar and select Lambda to open the service console.
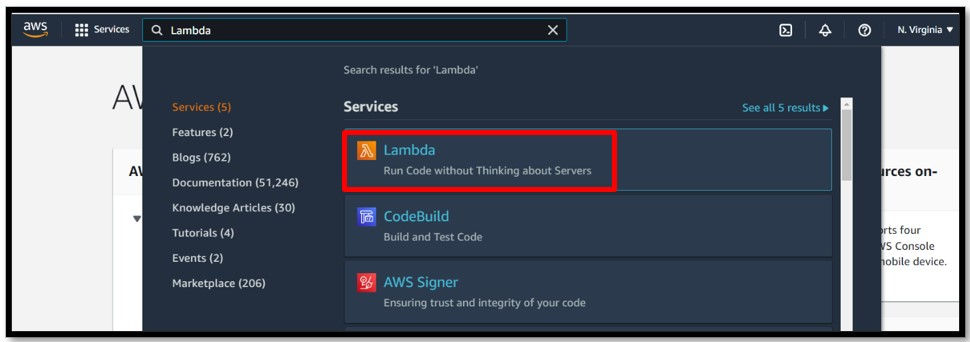
Create a Lambda function by clicking on ‘Create Function’.
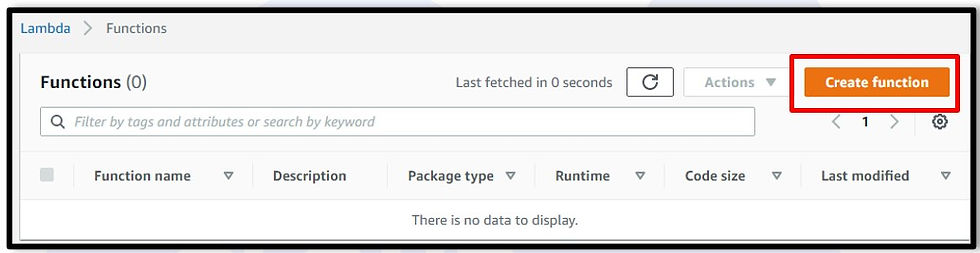
For Name, type MockAPIFunction.
For Runtime, choose Python 3.9.
For Architecture, choose arm64.
In Change default execution role
For Role, select use an existing role. Existing role: lambda_basic_execution
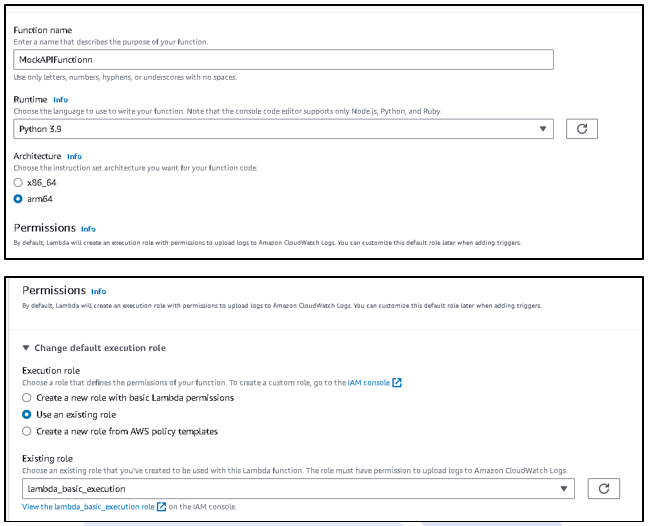
Click Create function.
On the MockAPIFunction screen, scroll down to the Function code section. In the code window, replace all of the code with the following, and choose Save.
class TooManyRequestsException(Exception): pass
class ServerUnavailableException(Exception): pass
class UnknownException(Exception): pass
def lambda_handler(event, context):
statuscode = event["statuscode"]
if statuscode == "429":
raise TooManyRequestsException('429 Too Many Requests')
elif statuscode == "503":
raise ServerUnavailableException('503 Server Unavailable')
elif statuscode == "200":
return '200 OK'
else:
raise UnknownException('Unknown error')
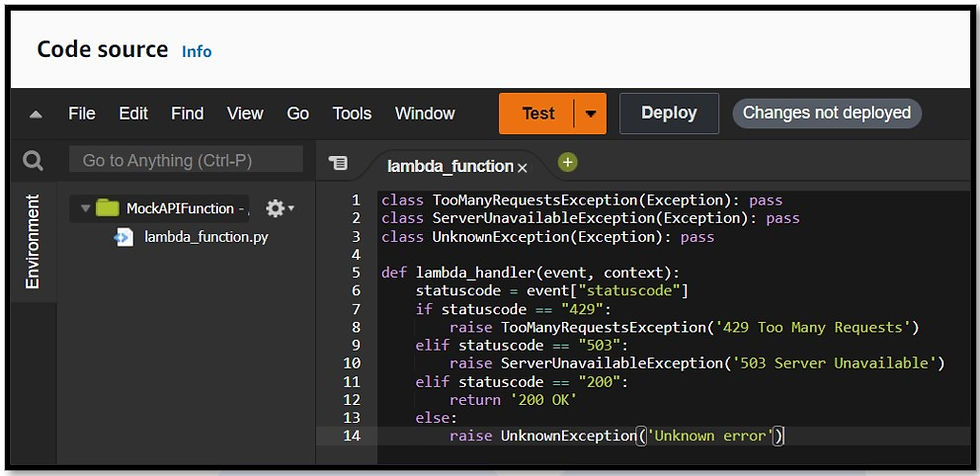
Once the Lambda function is created, scroll to the top of the window and note its Amazon Resource Name (ARN).
Step 3. Create a Step Functions State Machine
Open the AWS Step Function console, Click on Create State Machine.

Choose authoring method: Write your workflow in code
Type: Standard
Replace the contents of the State machine definition section with the following code:
{
"Comment": "An example of using retry and catch to handle API responses",
"StartAt": "Call API",
"States": {
"Call API": {
"Type": "Task",
"Resource": "lambda function arn paste here",
"Next" : "OK",
"Comment": "Catch a 429 (Too many requests) API exception, and resubmit the failed request in a rate-limiting fashion.",
"Retry" : [ {
"ErrorEquals": [ "TooManyRequestsException" ],
"IntervalSeconds": 1,
"MaxAttempts": 2
} ],
"Catch": [
{
"ErrorEquals": ["TooManyRequestsException"],
"Next": "Wait and Try Later"
}, {
"ErrorEquals": ["ServerUnavailableException"],
"Next": "Server Unavailable"
}, {
"ErrorEquals": ["States.ALL"],
"Next": "Catch All"
}
]
},
"Wait and Try Later": {
"Type": "Wait",
"Seconds" : 1,
"Next" : "Change to 200"
},
"Server Unavailable": {
"Type": "Fail",
"Error":"ServerUnavailable",
"Cause": "The server is currently unable to handle the request."
},
"Catch All": {
"Type": "Fail",
"Cause": "Unknown error!",
"Error": "An error of unknown type occurred"
},
"Change to 200": {
"Type": "Pass",
"Result": {"statuscode" :"200"} ,
"Next": "Call API"
},
"OK": {
"Type": "Pass",
"Result": "The request has succeeded.",
"End": true
}
}
}
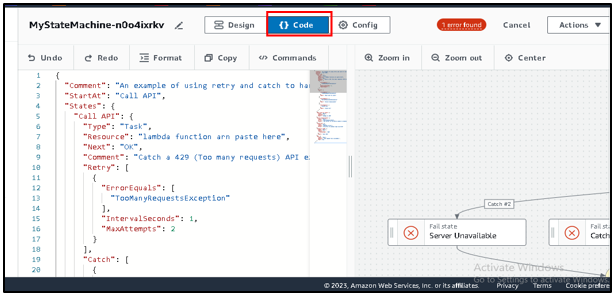
c. Find the “Resource” line in the “Call API” Task state (line 7). To update this ARN to the ARN of the mock API Lambda function you just created, click on the ARN text and then select the ARN from the list.

Create a state machine, select Author from scratch, state machine name: MyAPIStateMachine, and then select I will use an existing role.
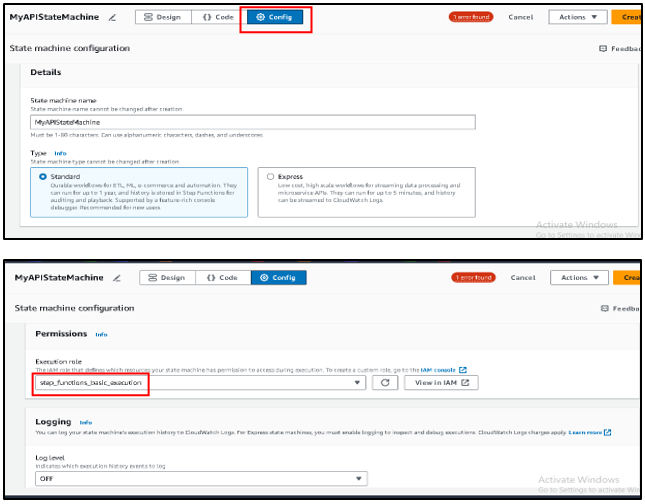
Create State Machines.
Also Deploy lambda function.

Step 4. Test your Error Handling Workflow
Click on Start execution.

A new execution dialog box appears, Replace the existing text with the code below, then choose Start execution:
{
"statuscode": "200"
}
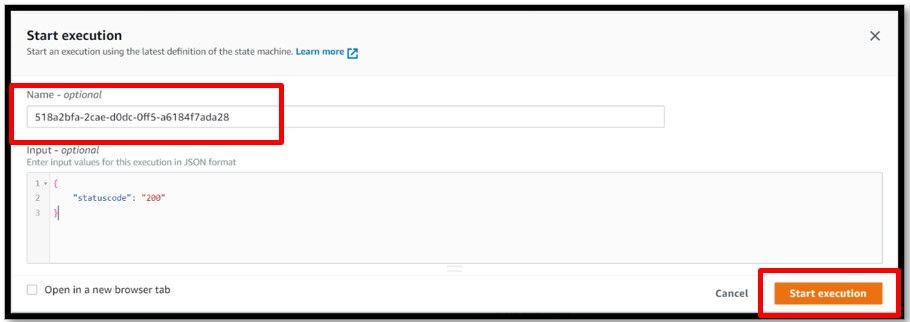
On the Execution details screen, click Input to see the input. Next, click Output to view the result of state machine execution. You can see that the workflow interpreted statuscode 200 as a successful API call.
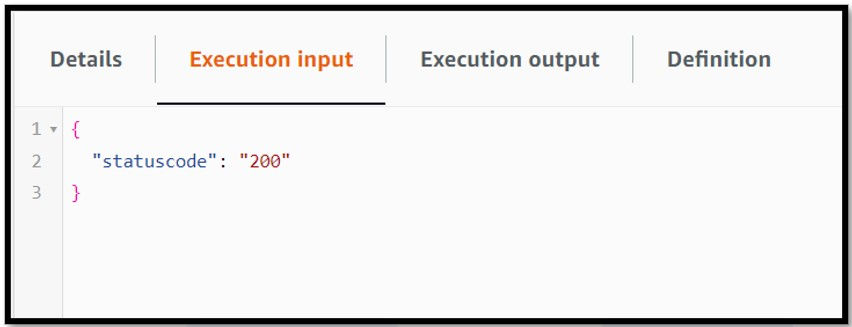

This Task state successfully invoked mock API Lambda function with the input you provided, and captured the output of that Lambda function, “200 OK”.
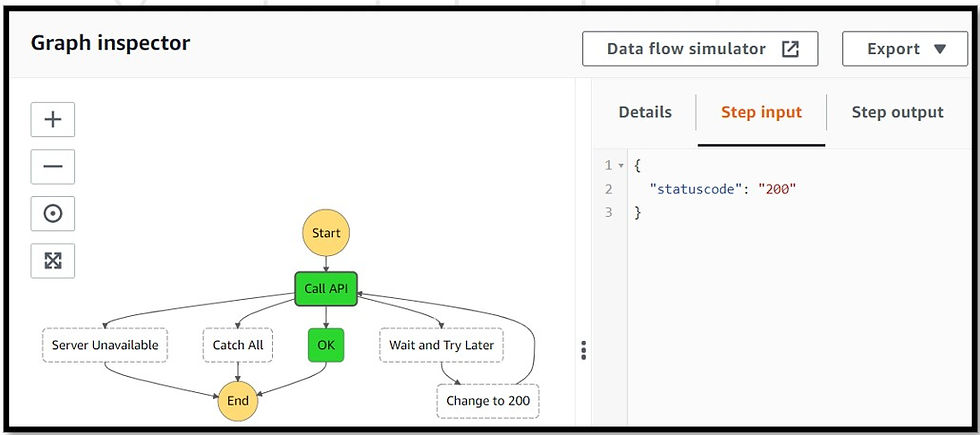
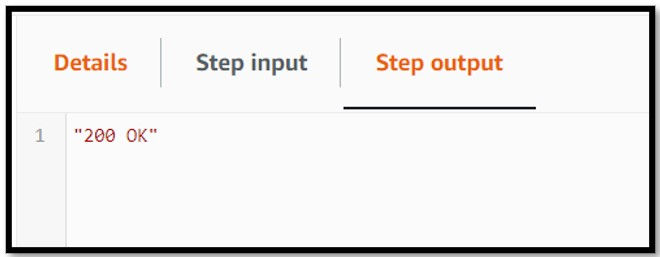
Next, click on the "OK" Task state in the visual workflow.
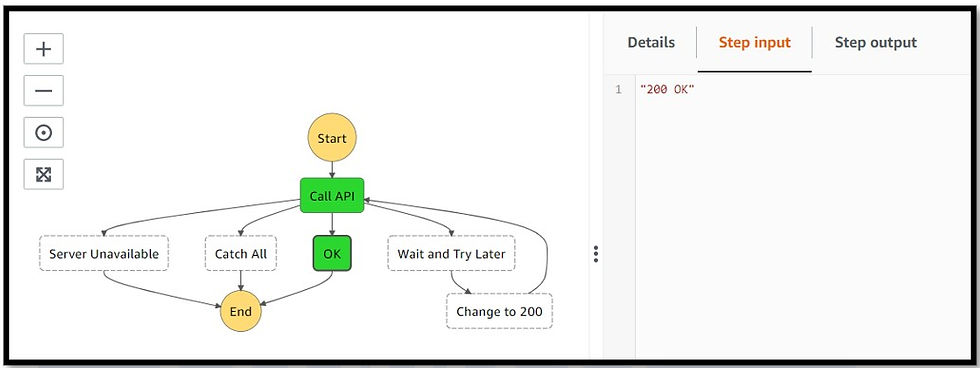
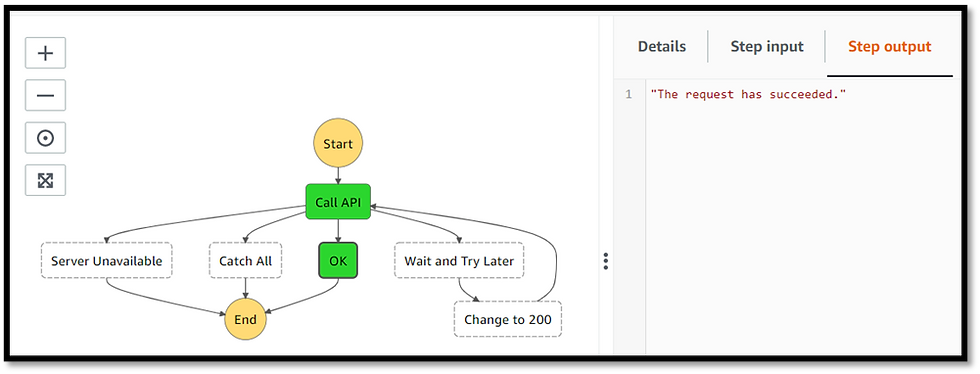
Step 5. Inspect the Execution of your State Machine
Scroll to the top of the Execution details screen and click on MyAPIStateMachine.
Click on Start execution again, and this time provide the following input and then click Start execution.
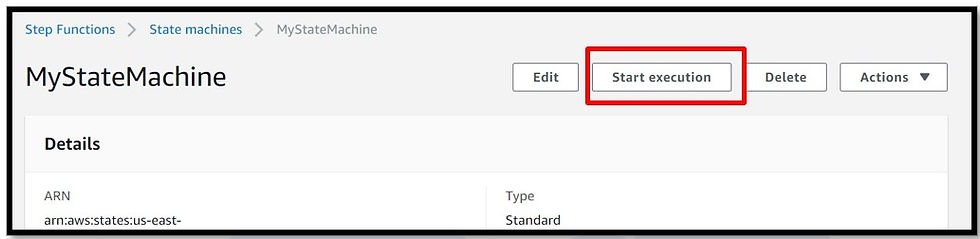
Click on Start execution again, and this time provide the following input and then click Start execution.
{
“statuscode”: “503”
}
In the Execution event history section, expand each execution step to confirm that your workflow behaved as expected.
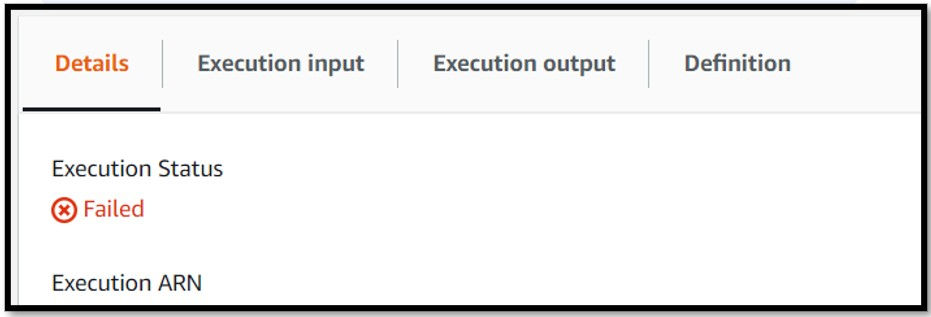


Next, simulate a 429 exception. Scroll to the top of the Execution details screen and click on MyAPIStateMachine. Click on Start execution, provide the following input, and click Start execution.
{
“statuscode”: “429”
}

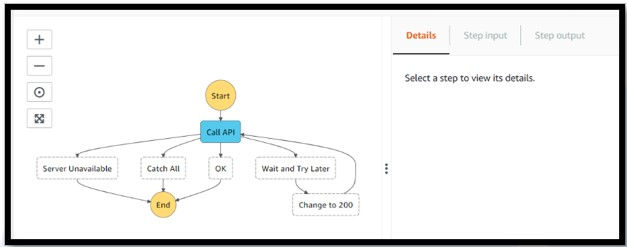
Next, the Wait state used brute force to change the response code to 200, and your workflow completed execution successfully.
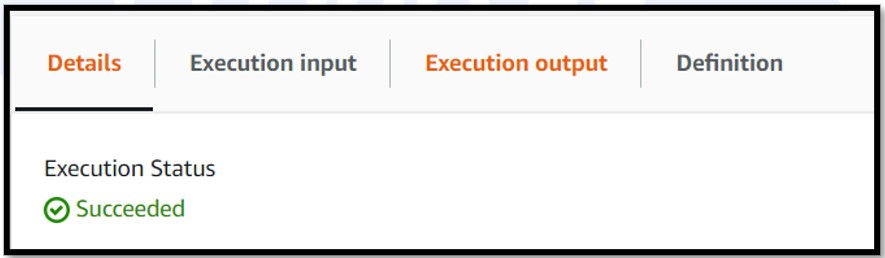
Note: If you no longer need the resources, you may delete the Lambda Function, the state machine, IAM role
Was this document helpful? How can we make this document better? Please provide your insights. You can download PDF version for reference.
We provide the best AWS training from Pune, India.
For aws certification contact us now.
very useful sir
Easy to understand
Easy to follow
Good blog
Easy to understand the concept